Receive incoming SMS
If an incoming delivery URL is specified in account settings, incoming SMS messages will be sent to that URL whenever they are available. The contents of these SMS messages are described below.
Our system sends the report using POST and expects a 200 OK response if the report is delivered correctly. If any other status (ie an error status) is received, the report will be sent again at a later time.
You manage your callback url in the user portal https://portal.ip1.net/accounts/ → Settings for incoming SMS (See image below)
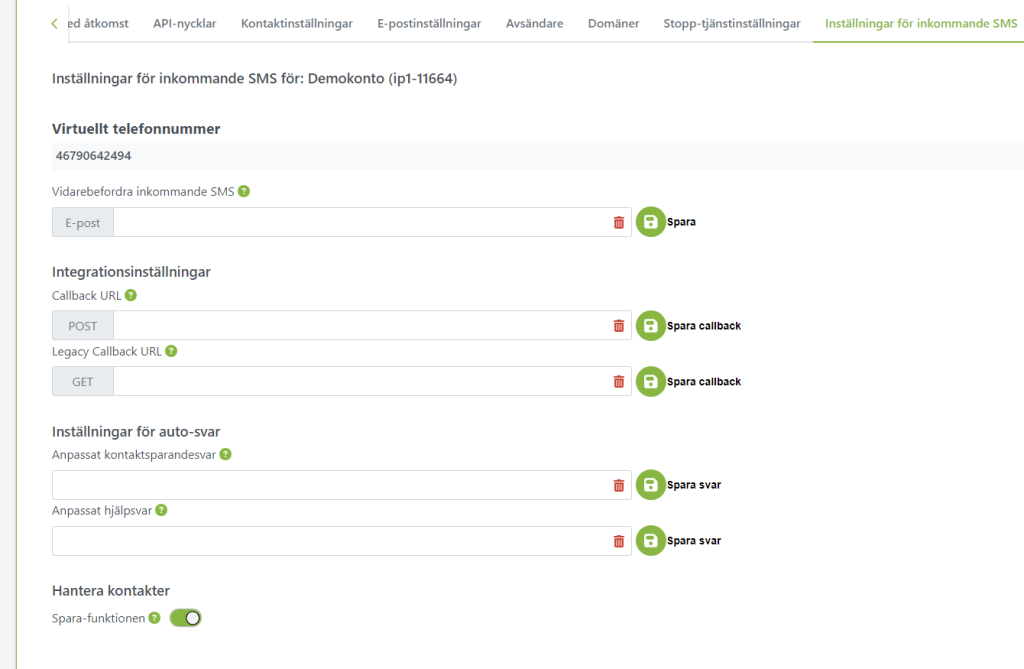
Report Payload
{
"id": "5c613848879973045cf39ac4",
"batchId": "5c613848879973045cf39ac3",
"owner": "ip1-XXXXX",
"sender": "TestNOS",
"recipient": "456189040623",
"body": "Hi my name is earl",
"direction": "mt",
"segments": 1,
"type":"SMS",
"datacoding": "GSM",
"priority": 1,
"statuses": [
{
"created": "2018-10-23T17:43:21Z",
"code": 201,
"duration": 1
}
],
"modified": "2018-10-23T17:43:19Z",
"mcc": "431",
"mnc": "20"
}
id
A unique ID for the specific message.
batchId
An ID for all the messages received in the same request as this message. This is usually just the one.
owner
The account ID of the SMS account that owns the message (e.g. you).
sender
The sender ID of the message's originator.
recipient
The recipient of the message (e.g. your virtual number).
body
The message contents.
direction
Determines whether the message was sent or received by our systems. Should always be mo
here.
mt
an acronym for mobile terminated.mo
an acronym for mobile originated.
segments
In case the message contains more characters than one sms can hold the message will be split into multiple sms also known as concatenated sms. This property indicates how many sms the message needs to be sent.
datacoding
Specifies the datacoding scheme used to send the message in question.
priority
The priority in which the message was sent. Priority 1 is the default and is the lowest priority that is available. Priority 2 is the higher priority.
statuses
An array of status updates.
- statuses[].created
- when the status was added
- statuses[].code
- The specific status code. More may be added in the future
- statuses[].duration
- Tells whether this is the last status update or if there may be more status updates to come
Incoming messages only have one provided status as it entered our systems from outside. For more information regarding our status codes for messages please see our section about Status codes.
modified
When the SMS was last updated.
mcc
The country part of the leaf operator may be specified here if it's provided by the upstream carrier.
MCC
an acronym for Mobile Country Code
mnc
The network part of the leaf operator may be specified here if it's provided by the upstream carrier.
MNC
an acronym for Mobile Network Code
Code example
Below you will find a code example for a callback for incoming SMS traffic.
Callback for incoming SMS C#
[Route("callback")]
[ApiController]
public class CallbackController : ControllerBase
{
private readonly SqlConnection Connection;
public CallbackController()
{
Connection = new SqlConnection("Connection String");
Connection.Open();
}
[HttpPost("")]
public ActionResult HandleCallback(IncommingSms request)
{
using (SqlCommand cmd = new SqlCommand("INSERT INTO [Messaging].[SmsMessage] ([Sender],[Body]) VALUES (@sender, @body)", Connection))
{
cmd.Parameters.AddWithValue("@sender", request.Sender);
cmd.Parameters.AddWithValue("@body", request.Body);
int result = cmd.ExecuteNonQuery();
}
return Ok();
}
}
public class IncommingSms
{
public string Id { get; set; }
public string BatchId { get; set; }
public string Owner { get; set; }
public string Sender { get; set; }
public string Recipient { get; set; }
public string Body { get; set; }
public string Direction { get; set; }
public int Segments { get; set; }
public string Type { get; set; }
public string Datacoding { get; set; }
public int Priority { get; set; }
public List Statuses { get; set; }
public DateTime Modified { get; set; }
public string Mcc { get; set; }
public string Mnc { get; set; }
}
public class Status
{
public DateTime Created { get; set; }
public int Code { get; set; }
public int Duration { get; set; }
}